Quick C# fundamentals revision
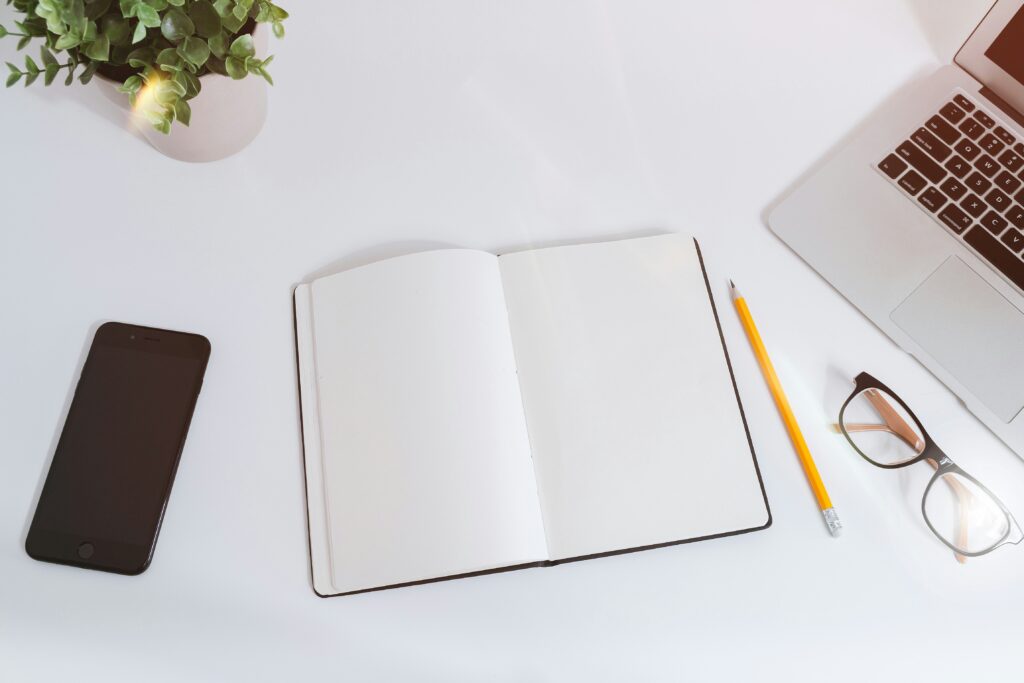
This is a quick overview of C# concepts for interview preparations.
.NET (Framework, Core) and C#
.Net Framework is a framework created by Microsoft to design applications to be run on the Windows platform. The applications can be built using many languages (VB.Net, F# etc.) and C# is one such programming language.
The framework (.NET) specifically for the Windows platform is called .NET Framework. Various versions of .NET Framework exists with the .NET Framework 4.8.1 being the last. Microsoft’s strategy for .NET changed after 2015 to support cross platform development and a new framework called .NET Core was designed. Until versions 3.1, the cross platform .NET was referred to as .NET Core <version> i.e., .Net Core 3.1 in this case. However, subsequently the word Core was dropped and it has been renamed to .Net <version>. There is no .NET 4 to avoid confusion with .NET Framework.
The framework that is the .NET on its own comprises two key components:
a) Common Language Runtime (CLR), and
b) Class library
Upon compilation, the C# code is transformed into IL (Intermediate Language) code. The common language runtime is an application in the memory that translates and runs the IL code on the machine. This process is called Just-In-Time (JIT) compilation. This is how the .NET framework supports multiple languages. The compilers of each supported language transforms the original source code into the same IL code. Thus the programmer doesn’t have to worry about compiling the C# code into the native machine code specific to each machine. If the machine has CLR, it can run the application.
.NET applications architecture
Any C# application consists of classes as the building blocks. A class is basically a bucket (or container) for data (or attributes) that describes the class and methods (or functions) that the class does. For example, a person class will have attributes such as name, age etc. and can perform actions such as say hi or walk.
A group of classes related with each other are bundled together under a namespace. This allows for better maintainability as the application grows.
An assembly (a dynamic link library (DLL) or an executable (EXE)) is a container of related namespaces and classes. It is a single unit of deployment of the .NET applications. DLL files can be reused across different programs and an EXE file can be executed.
Variables, Constants
Variables are names that points to a particular location in memory. Constants are immutable i.e. their values cannot be changed while the program is running.
int year;
int month = 5; // declare variables using camelCase
const int DateOfBirthYear = 2000; // declare constants using PascalCase
Primitive types and non-primitive (derived) types
These are the most used primitive types:
a) For integers, use – byte, short, int.
b) For real numbers use – float, double, decimal. The numbers need to suffixed by f for float and m for decimal.
c) For characters use char.
d) For booleans use bool.
// real numbers declaration example
float stockPrice = 400.1f;
double stockPrice = 400.1;
decimal stockPrice = 400.1m;
A variable is accessible within the scope it has been defined under. This is set by the curly braces {}.