Object Oriented Programming (OOP)
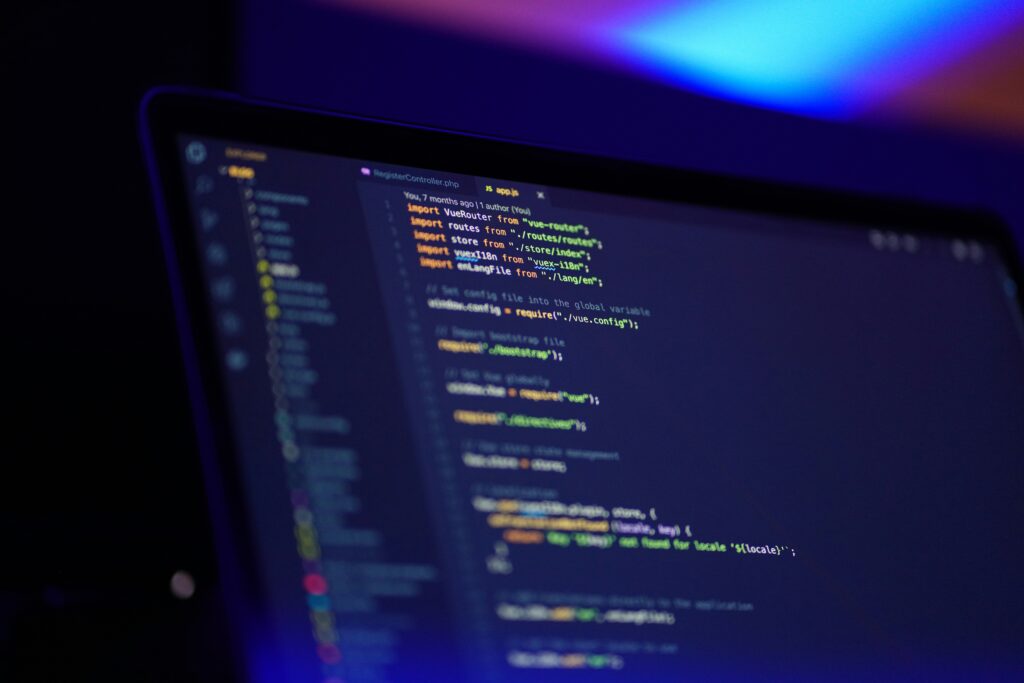
Classes, Interfaces and concepts such as encapsulation, abstraction, inheritance and polymorphism – it all started with what really is object oriented programming.
Our body at its core is comprised of cells. Group of cells together form an organ and group of organs together form our body. The organs communicate with each other to achieve a particular function. Similar was the idea with the object oriented programming. The classes i.e. cells would communicate with each other to achieve a particular function. An organ doesn’t need to know the underlying structural composition of the other organ. It just sends a message and waits for the response.
So, what is a class?
Think of class as the lowest level building block. It consists of fields or attributes for storing data and methods or functions for performing operations.
For example, suppose we want to create an Order class for placing a stock order in the market. An order generally has the following attributes:
Quantity | Integer or decimal value representing the number of units |
Security | The identifier (symbol or ticker) of the security or instrument |
Action | Either buy or sell |
Type | Market or Limit |
So we can have enums representing action and type and a class representing an order as below.
public class Order
{
public int Quantity { get; private set; }
public string Security { get; private set; }
public OrderAction OrderAction { get; private set; }
public OrderType OrderType { get; private set; }
public Order(int quantity, string security, OrderAction orderAction, OrderType orderType)
{
Quantity = quantity;
Security = security;
OrderAction = orderAction;
OrderType = orderType;
}
}
public enum OrderAction
{
Buy,
Sell
}
public enum OrderType
{
Market,
Limit
}
Note that we are using a constructor (the method that gets called when creating a new instance of the class) to initialize the order i.e. we need the above four properties to create an order.
So, if we want to have a buy market order of 1 unit for Tesla (ticker TSLA) stock, we can have it as below.
var order = new Order(1, "TSLA", OrderAction.Buy, OrderType.Market);
Now, what is an Interface?
Consider Interfaces to be the contracts which each class that implements it has to satisfy. Programming against interfaces instead of directly using classes helps to build a loosely coupled application.